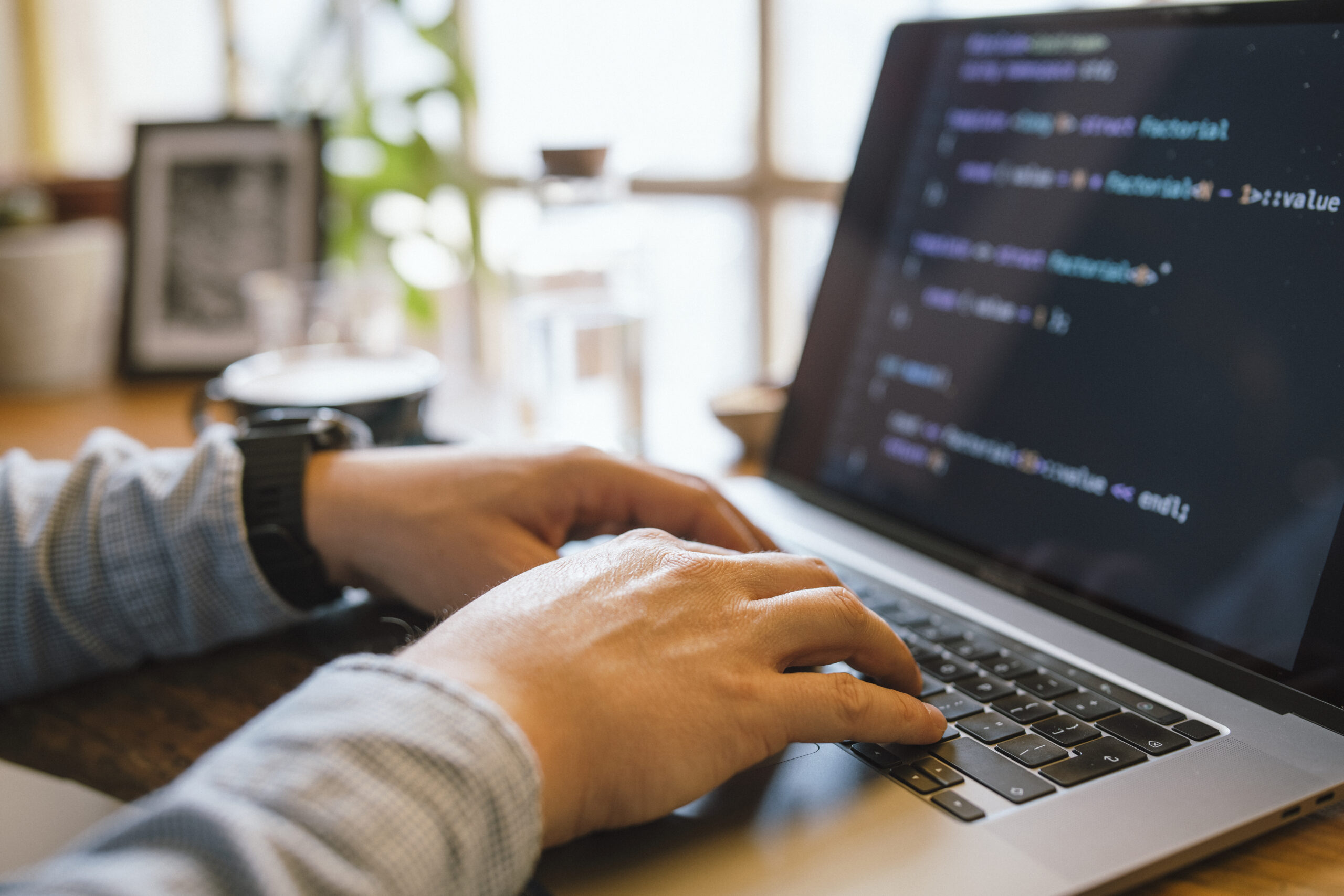
Debugging is One of the more essential — but typically forgotten — skills inside a developer’s toolkit. It is not nearly repairing broken code; it’s about comprehension how and why points go Completely wrong, and Discovering to think methodically to solve problems efficiently. Whether or not you're a beginner or a seasoned developer, sharpening your debugging skills can preserve hrs of annoyance and radically help your efficiency. Here i will discuss quite a few procedures that will help builders amount up their debugging video game by me, Gustavo Woltmann.
Learn Your Applications
Among the quickest techniques developers can elevate their debugging expertise is by mastering the instruments they use every single day. Even though producing code is one Element of enhancement, recognizing how to connect with it properly in the course of execution is equally important. Modern-day advancement environments come Geared up with impressive debugging abilities — but quite a few developers only scratch the surface of what these resources can do.
Choose, by way of example, an Built-in Growth Setting (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These tools assist you to set breakpoints, inspect the worth of variables at runtime, move as a result of code line by line, and in some cases modify code around the fly. When made use of effectively, they Allow you to notice specifically how your code behaves all through execution, that's invaluable for monitoring down elusive bugs.
Browser developer tools, for instance Chrome DevTools, are indispensable for front-end builders. They let you inspect the DOM, monitor network requests, see serious-time efficiency metrics, and debug JavaScript from the browser. Mastering the console, resources, and community tabs can flip disheartening UI difficulties into manageable responsibilities.
For backend or program-amount developers, equipment like GDB (GNU Debugger), Valgrind, or LLDB offer deep Command over managing processes and memory administration. Studying these applications could possibly have a steeper Understanding curve but pays off when debugging performance problems, memory leaks, or segmentation faults.
Beyond your IDE or debugger, turn out to be comfortable with Model Management methods like Git to comprehend code record, uncover the exact minute bugs were being introduced, and isolate problematic modifications.
Eventually, mastering your applications means going over and above default configurations and shortcuts — it’s about acquiring an intimate understanding of your advancement setting making sure that when challenges crop up, you’re not shed at the hours of darkness. The greater you know your tools, the more time you can spend solving the actual problem rather than fumbling through the process.
Reproduce the challenge
Among the most important — and infrequently neglected — measures in successful debugging is reproducing the trouble. Just before jumping in to the code or generating guesses, developers need to produce a reliable setting or situation exactly where the bug reliably seems. Devoid of reproducibility, repairing a bug gets to be a game of prospect, typically leading to squandered time and fragile code alterations.
The first step in reproducing a dilemma is accumulating as much context as possible. Talk to inquiries like: What actions triggered The problem? Which atmosphere was it in — development, staging, or generation? Are there any logs, screenshots, or mistake messages? The greater detail you may have, the less difficult it gets to be to isolate the precise ailments below which the bug takes place.
After you’ve collected plenty of info, try to recreate the situation in your local environment. This might mean inputting precisely the same info, simulating identical user interactions, or mimicking process states. If the issue appears intermittently, take into consideration composing automatic tests that replicate the edge conditions or state transitions included. These checks not just enable expose the problem but in addition reduce regressions Later on.
From time to time, The difficulty might be setting-unique — it might take place only on selected functioning methods, browsers, or beneath unique configurations. Making use of equipment like Digital equipment, containerization (e.g., Docker), or cross-browser tests platforms may be instrumental in replicating these kinds of bugs.
Reproducing the situation isn’t simply a step — it’s a attitude. It calls for endurance, observation, and also a methodical solution. But once you can regularly recreate the bug, you are previously midway to repairing it. That has a reproducible state of affairs, You should use your debugging equipment far more proficiently, exam opportunity fixes properly, and communicate much more clearly along with your group or consumers. It turns an abstract complaint into a concrete challenge — Which’s wherever builders thrive.
Go through and Realize the Error Messages
Mistake messages will often be the most beneficial clues a developer has when a little something goes Erroneous. In lieu of observing them as aggravating interruptions, developers should master to deal with error messages as direct communications within the process. They typically let you know precisely what transpired, wherever it took place, and occasionally even why it happened — if you know the way to interpret them.
Start out by reading through the message diligently As well as in complete. Lots of builders, especially when less than time strain, glance at the main line and quickly start off creating assumptions. But further inside the mistake stack or logs may possibly lie the accurate root induce. Don’t just copy and paste mistake messages into serps — study and have an understanding of them 1st.
Break the error down into parts. Could it be a syntax mistake, a runtime exception, or even a logic error? Does it issue to a particular file and line selection? What module or perform brought on it? These concerns can tutorial your investigation and stage you towards the liable code.
It’s also valuable to understand the terminology with the programming language or framework you’re utilizing. Mistake messages in languages like Python, JavaScript, or Java often stick to predictable styles, and Studying to acknowledge these can drastically quicken your debugging course of action.
Some errors are vague or generic, As well as in Those people instances, it’s critical to look at the context in which the error transpired. Look at associated log entries, enter values, and up to date variations within the codebase.
Don’t forget about compiler or linter warnings both. These normally precede bigger concerns and supply hints about probable bugs.
Ultimately, error messages aren't your enemies—they’re your guides. Finding out to interpret them the right way turns chaos into clarity, helping you pinpoint problems more quickly, lessen debugging time, and turn into a additional economical and self-assured developer.
Use Logging Sensibly
Logging is one of the most potent resources within a developer’s debugging toolkit. When employed properly, it provides true-time insights into how an application behaves, supporting you fully grasp what’s occurring beneath the hood while not having to pause execution or action from the code line by line.
A fantastic logging approach commences with being aware of what to log and at what stage. Frequent logging amounts contain DEBUG, Information, WARN, Mistake, and Lethal. Use DEBUG for specific diagnostic data for the duration of advancement, Information for common events (like successful get started-ups), Alert for prospective concerns that don’t break the applying, Mistake for real issues, and Lethal if the program can’t continue.
Stay away from flooding your logs with excessive or irrelevant details. An excessive amount logging can obscure crucial messages and slow down your system. Center on essential occasions, point out alterations, input/output values, and significant selection points as part of your code.
Format your log messages Evidently and constantly. Include context, for instance timestamps, request IDs, and performance names, so it’s easier to trace difficulties in distributed devices or multi-threaded environments. Structured logging (e.g., JSON logs) could make it even much easier to parse and filter logs programmatically.
In the course of debugging, logs Permit you to monitor how variables evolve, what disorders are satisfied, and what branches of logic are executed—all without halting the program. They’re Primarily precious in production environments the place stepping through code isn’t attainable.
Additionally, use logging frameworks and equipment (like Log4j, Winston, or Python’s logging module) that assistance log rotation, filtering, and integration with checking dashboards.
Ultimately, smart logging is about equilibrium and clarity. Having a very well-thought-out logging technique, you can decrease the time it will require to identify concerns, attain deeper visibility into your programs, and Increase the overall maintainability and dependability of your respective code.
Imagine Like a Detective
Debugging is not only a complex endeavor—it's a type of investigation. To proficiently detect and repair bugs, developers have to solution the process like a detective fixing a thriller. This way of thinking allows break down complicated concerns into workable parts and stick to clues logically to uncover the basis lead to.
Get started by accumulating proof. Think about the symptoms of the issue: error messages, incorrect output, or functionality troubles. The same as a detective surveys against the law scene, accumulate just as much related info as you'll be able to with no jumping to conclusions. Use logs, examination circumstances, and user reviews to piece with each other a clear picture of what’s happening.
Next, form hypotheses. Ask yourself: What could be causing this actions? Have any variations a short while ago been built to your codebase? Has this situation transpired just before beneath comparable circumstances? The intention will be to slim down opportunities and recognize prospective culprits.
Then, check your theories systematically. Try to recreate the condition in a very controlled surroundings. In the event you suspect a selected purpose or element, isolate it and validate if The problem persists. Like a detective conducting interviews, check with your code inquiries and let the final results direct you nearer to the truth.
Pay near interest to compact information. Bugs often cover inside the the very least anticipated places—just like a lacking semicolon, an off-by-one particular mistake, or possibly a race condition. Be extensive and individual, resisting the urge to patch The difficulty with no fully knowledge it. Temporary fixes may well hide the true problem, only for it to resurface afterwards.
Finally, retain notes on what you experimented with and learned. Just as detectives log their investigations, documenting your debugging course of action can save time for foreseeable future issues and aid Some others comprehend your reasoning.
By contemplating similar to a detective, developers can sharpen their analytical expertise, tactic problems methodically, and grow to be simpler at uncovering concealed issues in sophisticated devices.
Write Tests
Composing assessments is among the simplest methods to help your debugging abilities and All round growth performance. Tests not just aid catch bugs early but in addition function a security Web that offers you assurance when making modifications in your codebase. A properly-examined application is simpler to debug since it lets you pinpoint just wherever and when a challenge takes place.
Begin with device exams, which target specific features or modules. These modest, isolated exams can swiftly reveal regardless of whether a particular piece of logic is Operating as expected. Any time a exam fails, you straight away know wherever to glance, drastically minimizing time invested debugging. Unit checks are In particular handy for catching regression bugs—troubles that reappear immediately after Formerly becoming fixed.
Future, combine integration exams and end-to-end assessments into your workflow. These aid make certain that various aspects of your application function alongside one another efficiently. They’re specifically useful for catching bugs that come about in sophisticated methods with various elements or solutions interacting. If a little something breaks, your exams can show you which Section of the pipeline failed and underneath what circumstances.
Crafting exams also forces you to definitely Feel critically regarding your code. To test a aspect appropriately, you'll need to be aware of its inputs, anticipated outputs, and edge scenarios. This degree of being familiar with By natural means potential customers to better code framework and fewer bugs.
When debugging a problem, crafting a failing check that reproduces the bug can be a strong starting point. Once the examination fails continuously, you'll be able to center on fixing the bug and observe your exam pass when The problem is solved. This solution ensures that precisely the same bug doesn’t return Down the road.
In brief, composing checks turns debugging from the irritating guessing match right into a structured and predictable procedure—supporting you capture extra bugs, more quickly and a lot more reliably.
Choose Breaks
When debugging click here a tricky problem, it’s straightforward to be immersed in the situation—gazing your screen for hours, attempting Remedy soon after Option. But One of the more underrated debugging tools is simply stepping away. Taking breaks assists you reset your thoughts, minimize disappointment, and sometimes see The problem from a new viewpoint.
When you're also close to the code for also prolonged, cognitive tiredness sets in. You could possibly start off overlooking clear problems or misreading code that you just wrote just hrs earlier. Within this state, your Mind results in being fewer successful at challenge-fixing. A short walk, a espresso crack, as well as switching to a distinct activity for 10–15 minutes can refresh your aim. Quite a few builders report locating the root of a dilemma when they've taken time and energy to disconnect, letting their subconscious work during the qualifications.
Breaks also aid stop burnout, especially all through extended debugging sessions. Sitting down before a screen, mentally trapped, is not merely unproductive but additionally draining. Stepping absent lets you return with renewed Power in addition to a clearer frame of mind. You could suddenly detect a missing semicolon, a logic flaw, or simply a misplaced variable that eluded you ahead of.
If you’re caught, a great general guideline is usually to set a timer—debug actively for 45–sixty minutes, then take a 5–ten minute crack. Use that time to maneuver around, stretch, or do something unrelated to code. It might experience counterintuitive, Specially less than restricted deadlines, but it really truly causes more quickly and more practical debugging in the long run.
Briefly, having breaks just isn't an indication of weak spot—it’s a smart approach. It presents your brain Room to breathe, increases your perspective, and will help you steer clear of the tunnel vision that often blocks your progress. Debugging is usually a mental puzzle, and rest is a component of resolving it.
Learn From Each Bug
Each bug you encounter is much more than simply A short lived setback—it's a chance to increase to be a developer. Whether or not it’s a syntax error, a logic flaw, or possibly a deep architectural difficulty, each one can instruct you some thing useful in case you go to the trouble to reflect and examine what went Mistaken.
Start out by inquiring you a few important queries when the bug is solved: What brought about it? Why did it go unnoticed? Could it are caught before with improved tactics like device tests, code assessments, or logging? The responses normally expose blind places as part of your workflow or being familiar with and assist you Establish much better coding patterns going ahead.
Documenting bugs can even be a fantastic routine. Hold a developer journal or sustain a log where you note down bugs you’ve encountered, the way you solved them, and Anything you figured out. After some time, you’ll start to see patterns—recurring issues or common issues—you can proactively prevent.
In crew environments, sharing Everything you've discovered from the bug with the peers may be especially highly effective. No matter whether it’s through a Slack information, a brief create-up, or A fast expertise-sharing session, aiding Other people steer clear of the very same problem boosts workforce effectiveness and cultivates a stronger Mastering tradition.
More importantly, viewing bugs as classes shifts your mindset from irritation to curiosity. As an alternative to dreading bugs, you’ll start appreciating them as critical areas of your development journey. In spite of everything, a number of the most effective developers are usually not the ones who produce excellent code, but individuals that continually master from their blunders.
Eventually, Each and every bug you deal with adds a whole new layer to your ability established. So next time you squash a bug, take a minute to reflect—you’ll arrive absent a smarter, more capable developer as a consequence of it.
Summary
Bettering your debugging techniques takes time, follow, and tolerance — but the payoff is big. It would make you a far more effective, self-confident, and able developer. Another time you're knee-deep within a mysterious bug, recall: debugging isn’t a chore — it’s a possibility to become far better at That which you do.